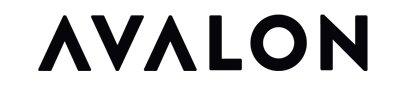
The safe post-production pipeline
Avalon¶
Welcome to the library documentation for Avalon, the safe post-production pipeline for film and games.
Library Summary
avalon.api |
Public application programming interface |
avalon.pipeline |
Core pipeline functionality |
avalon.schema |
Wrapper around jsonschema |
avalon.inventory |
Utilities for interacting with database through the Inventory API |
avalon.io |
Wrapper around interactions with the database |
avalon.lib |
Helper functions |
API¶
Public application programming interface
The following members are public and reliable. That is to say, anything not defined here is internal and likely unreliable for use outside of the codebase itself.
-
avalon.api.
install
(host)¶ Install host into the running Python session.
Parameters: host (module) – A Python module containing the Avalon avalon host-interface.
-
avalon.api.
uninstall
()¶ Undo all of what install() did
-
class
avalon.api.
Loader
(context)¶ Load representation into host application
Parameters: - context (dict) – avalon-core:context-1.0
- name (str, optional) – Use pre-defined name
- namespace (str, optional) – Use pre-defined namespace
New in version 4.0: This class was introduced
-
load
(context, name=None, namespace=None, options=None)¶ Load asset via database
Parameters: - context (dict) – Full parenthood of representation to load
- name (str, optional) – Use pre-defined name
- namespace (str, optional) – Use pre-defined namespace
- options (dict, optional) – Additional settings dictionary
-
remove
(container)¶ Remove a container
Parameters: (avalon-core (container) – container-1.0): Container to remove, from host.ls(). Returns: Whether the container was deleted Return type: bool
-
update
(container, representation)¶ Update container to representation
Parameters: - (avalon-core (container) – container-1.0): Container to update, from host.ls().
- representation (dict) – Update the container to this representation.
-
class
avalon.api.
Creator
(name, asset, options=None, data=None)¶ Determine how assets are created
-
class
avalon.api.
Action
¶ A custom action available
-
is_compatible
(session)¶ Return whether the class is compatible with the Session.
-
-
class
avalon.api.
InventoryAction
¶ A custom action for the scene inventory tool
If registered the action will be visible in the Right Mouse Button menu under the submenu “Actions”.
-
static
is_compatible
(container)¶ Override function in a custom class
This method is specifically used to ensure the action can operate on the container.
Parameters: container (dict) – the data of a loaded asset, see host.ls() Returns: bool
-
process
(containers)¶ Override function in a custom class
This method will receive all containers even those which are incompatible. It is advised to create a small filter along the lines of this example:
valid_containers = filter(self.is_compatible(c) for c in containers)
The return value will need to be a True-ish value to trigger the data_changed signal in order to refresh the view.
You can return a list of container names to trigger GUI to select treeview items.
- You can return a dict to carry extra GUI options. For example:
- {
“objectNames”: [container names…], “options”: {“mode”: “toggle”,
“clear”: False}
}
- Currently workable GUI options are:
- clear (bool): Clear current selection before selecting by action.
- Default True.
- mode (str): selection mode, use one of these:
- “select”, “deselect”, “toggle”. Default is “select”.
Parameters: containers (list) – list of dictionaries Returns: bool, list or dict
-
static
-
class
avalon.api.
Application
¶ Default application launcher
This is a convenience application Action that when “config” refers to a parsed application .toml this can launch the application.
-
environ
(session)¶ Build application environment
-
initialize
(environment)¶ Initialize work directory
-
is_compatible
(session)¶ Return whether the class is compatible with the Session.
-
process
(session, **kwargs)¶ Process the full Application action
-
-
avalon.api.
discover
(superclass)¶ Find and return subclasses of superclass
-
avalon.api.
on
(event, callback)¶ Call callback on event
Register callback to be run when event occurs.
Example
>>> def on_init(): ... print("Init happened") ... >>> on("init", on_init) >>> del on_init
Parameters: - event (str) – Name of event
- callback (callable) – Any callable
-
avalon.api.
after
(event, callback)¶ Convenience to on() for after-events
-
avalon.api.
before
(event, callback)¶ Convenience to on() for before-events
-
avalon.api.
emit
(event, args=None)¶ Trigger an event
Example
>>> def on_init(): ... print("Init happened") ... >>> on("init", on_init) >>> emit("init") Init happened >>> del on_init
Parameters: - event (str) – Name of event
- args (list, optional) – List of arguments passed to callback
-
avalon.api.
publish
()¶ Shorthand to publish from within host
-
avalon.api.
create
(name, asset, family, options=None, data=None)¶ Create a new instance
Associate nodes with a subset and family. These nodes are later validated, according to their family, and integrated into the shared environment, relative their subset.
Data relative each family, along with default data, are imprinted into the resulting objectSet. This data is later used by extractors and finally asset browsers to help identify the origin of the asset.
Parameters: - name (str) – Name of subset
- asset (str) – Name of asset
- family (str) – Name of family
- options (dict, optional) – Additional options from GUI
- data (dict, optional) – Additional data from GUI
Raises: - NameError on subset already exists –
- KeyError on invalid dynamic property –
- RuntimeError on host error –
Returns: Name of instance
-
avalon.api.
load
(Loader, representation, namespace=None, name=None, options=None, **kwargs)¶ Use Loader to load a representation.
Parameters: - Loader (Loader) – The loader class to trigger.
- representation (str or io.ObjectId or dict) – The representation id or full representation as returned by the database.
- namespace (str, Optional) – The namespace to assign. Defaults to None.
- name (str, Optional) – The name to assign. Defaults to subset name.
- options (dict, Optional) – Additional options to pass on to the loader.
Returns: The return of the loader.load() method.
Raises: IncompatibleLoaderError – When the loader is not compatible with the representation.
-
avalon.api.
update
(container, version=-1)¶ Update a container
-
avalon.api.
switch
(container, representation)¶ Switch a container to representation
Parameters: - container (dict) – container information
- representation (dict) – representation data from document
Returns: function call
-
avalon.api.
remove
(container)¶ Remove a container
-
avalon.api.
update_current_task
(task=None, asset=None, app=None)¶ Update active Session to a new task work area.
This updates the live Session to a different asset, task or app.
Parameters: - task (str) – The task to set.
- asset (str) – The asset to set.
- app (str) – The app to set.
Returns: The changed key, values in the current Session.
Return type: dict
-
avalon.api.
get_representation_path
(representation)¶ Get filename from representation document
There are three ways of getting the path from representation which are tried in following sequence until successful. 1. Get template from representation[‘data’][‘template’] and data from
representation[‘context’]. Then format template with the data.- Get template from project[‘config’] and format it with default data set
- Get representation[‘data’][‘path’] and use it directly
Parameters: representation (dict) – representation document from the database Returns: fullpath of the representation Return type: str
-
avalon.api.
loaders_from_representation
(loaders, representation)¶ Return all compatible loaders for a representation.
-
avalon.api.
register_host
(host)¶ Register a new host for the current process
Parameters: host (ModuleType) – A module implementing the Host API interface. See the Host API documentation for details on what is required, or browse the source code.
-
avalon.api.
register_plugin_path
(superclass, path)¶ Register a directory of one or more plug-ins
Parameters: - superclass (type) – Superclass of plug-ins to look for during discovery
- path (str) – Absolute path to directory in which to discover plug-ins
-
avalon.api.
register_plugin
(superclass, obj)¶ Register an individual obj of type superclass
Parameters: - superclass (type) – Superclass of plug-in
- obj (object) – Subclass of superclass
-
avalon.api.
register_root
(path)¶ Register currently active root
-
avalon.api.
registered_root
()¶ Return currently registered root
-
avalon.api.
registered_plugin_paths
()¶ Return all currently registered plug-in paths
-
avalon.api.
registered_host
()¶ Return currently registered host
-
avalon.api.
registered_config
()¶ Return currently registered config
-
avalon.api.
deregister_plugin
(superclass, plugin)¶ Oppsite of register_plugin()
-
avalon.api.
deregister_plugin_path
(superclass, path)¶ Oppsite of register_plugin_path()
-
avalon.api.
time
()¶ Return file-system safe string of current date and time
Pipeline¶
Core pipeline functionality
-
class
avalon.pipeline.
Action
¶ A custom action available
-
is_compatible
(session)¶ Return whether the class is compatible with the Session.
-
-
class
avalon.pipeline.
Application
¶ Default application launcher
This is a convenience application Action that when “config” refers to a parsed application .toml this can launch the application.
-
environ
(session)¶ Build application environment
-
initialize
(environment)¶ Initialize work directory
-
is_compatible
(session)¶ Return whether the class is compatible with the Session.
-
process
(session, **kwargs)¶ Process the full Application action
-
-
class
avalon.pipeline.
Creator
(name, asset, options=None, data=None)¶ Determine how assets are created
-
exception
avalon.pipeline.
IncompatibleLoaderError
¶ Error when Loader is incompatible with a representation.
-
class
avalon.pipeline.
InventoryAction
¶ A custom action for the scene inventory tool
If registered the action will be visible in the Right Mouse Button menu under the submenu “Actions”.
-
static
is_compatible
(container)¶ Override function in a custom class
This method is specifically used to ensure the action can operate on the container.
Parameters: container (dict) – the data of a loaded asset, see host.ls() Returns: bool
-
process
(containers)¶ Override function in a custom class
This method will receive all containers even those which are incompatible. It is advised to create a small filter along the lines of this example:
valid_containers = filter(self.is_compatible(c) for c in containers)
The return value will need to be a True-ish value to trigger the data_changed signal in order to refresh the view.
You can return a list of container names to trigger GUI to select treeview items.
- You can return a dict to carry extra GUI options. For example:
- {
“objectNames”: [container names…], “options”: {“mode”: “toggle”,
“clear”: False}
}
- Currently workable GUI options are:
- clear (bool): Clear current selection before selecting by action.
- Default True.
- mode (str): selection mode, use one of these:
- “select”, “deselect”, “toggle”. Default is “select”.
Parameters: containers (list) – list of dictionaries Returns: bool, list or dict
-
static
-
class
avalon.pipeline.
Loader
(context)¶ Load representation into host application
Parameters: - context (dict) – avalon-core:context-1.0
- name (str, optional) – Use pre-defined name
- namespace (str, optional) – Use pre-defined namespace
New in version 4.0: This class was introduced
-
load
(context, name=None, namespace=None, options=None)¶ Load asset via database
Parameters: - context (dict) – Full parenthood of representation to load
- name (str, optional) – Use pre-defined name
- namespace (str, optional) – Use pre-defined namespace
- options (dict, optional) – Additional settings dictionary
-
remove
(container)¶ Remove a container
Parameters: (avalon-core (container) – container-1.0): Container to remove, from host.ls(). Returns: Whether the container was deleted Return type: bool
-
update
(container, representation)¶ Update container to representation
Parameters: - (avalon-core (container) – container-1.0): Container to update, from host.ls().
- representation (dict) – Update the container to this representation.
-
avalon.pipeline.
after
(event, callback)¶ Convenience to on() for after-events
-
avalon.pipeline.
before
(event, callback)¶ Convenience to on() for before-events
-
avalon.pipeline.
compute_session_changes
(session, task=None, asset=None, app=None)¶ Compute the changes for a Session object on asset, task or app switch
This does NOT update the Session object, but returns the changes required for a valid update of the Session.
Parameters: - session (dict) – The initial session to compute changes to. This is required for computing the full Work Directory, as that also depends on the values that haven’t changed.
- task (str, Optional) – Name of task to switch to.
- asset (str or dict, Optional) – Name of asset to switch to. You can also directly provide the Asset dictionary as returned from the database to avoid an additional query. (optimization)
- app (str, Optional) – Name of app to switch to.
Returns: The required changes in the Session dictionary.
Return type: dict
-
avalon.pipeline.
create
(name, asset, family, options=None, data=None)¶ Create a new instance
Associate nodes with a subset and family. These nodes are later validated, according to their family, and integrated into the shared environment, relative their subset.
Data relative each family, along with default data, are imprinted into the resulting objectSet. This data is later used by extractors and finally asset browsers to help identify the origin of the asset.
Parameters: - name (str) – Name of subset
- asset (str) – Name of asset
- family (str) – Name of family
- options (dict, optional) – Additional options from GUI
- data (dict, optional) – Additional data from GUI
Raises: - NameError on subset already exists –
- KeyError on invalid dynamic property –
- RuntimeError on host error –
Returns: Name of instance
-
avalon.pipeline.
debug_host
()¶ A debug host, useful to debugging features that depend on a host
-
avalon.pipeline.
default_host
()¶ A default host, in place of anything better
This may be considered as reference for the interface a host must implement. It also ensures that the system runs, even when nothing is there to support it.
-
avalon.pipeline.
deregister_config
()¶ Undo register_config()
-
avalon.pipeline.
deregister_plugin
(superclass, plugin)¶ Oppsite of register_plugin()
-
avalon.pipeline.
deregister_plugin_path
(superclass, path)¶ Oppsite of register_plugin_path()
-
avalon.pipeline.
discover
(superclass)¶ Find and return subclasses of superclass
-
avalon.pipeline.
emit
(event, args=None)¶ Trigger an event
Example
>>> def on_init(): ... print("Init happened") ... >>> on("init", on_init) >>> emit("init") Init happened >>> del on_init
Parameters: - event (str) – Name of event
- args (list, optional) – List of arguments passed to callback
-
avalon.pipeline.
get_representation_context
(representation)¶ Return parenthood context for representation.
Parameters: representation (str or io.ObjectId or dict) – The representation id or full representation as returned by the database. Returns: The full representation context. Return type: dict
-
avalon.pipeline.
get_representation_path
(representation)¶ Get filename from representation document
There are three ways of getting the path from representation which are tried in following sequence until successful. 1. Get template from representation[‘data’][‘template’] and data from
representation[‘context’]. Then format template with the data.- Get template from project[‘config’] and format it with default data set
- Get representation[‘data’][‘path’] and use it directly
Parameters: representation (dict) – representation document from the database Returns: fullpath of the representation Return type: str
-
avalon.pipeline.
install
(host)¶ Install host into the running Python session.
Parameters: host (module) – A Python module containing the Avalon avalon host-interface.
-
avalon.pipeline.
is_compatible_loader
(Loader, context)¶ Return whether a loader is compatible with a context.
This checks the version’s families and the representation for the given Loader.
Returns: bool
-
avalon.pipeline.
is_installed
()¶ Return state of installation
Returns: True if installed, False otherwise
-
avalon.pipeline.
load
(Loader, representation, namespace=None, name=None, options=None, **kwargs)¶ Use Loader to load a representation.
Parameters: - Loader (Loader) – The loader class to trigger.
- representation (str or io.ObjectId or dict) – The representation id or full representation as returned by the database.
- namespace (str, Optional) – The namespace to assign. Defaults to None.
- name (str, Optional) – The name to assign. Defaults to subset name.
- options (dict, Optional) – Additional options to pass on to the loader.
Returns: The return of the loader.load() method.
Raises: IncompatibleLoaderError – When the loader is not compatible with the representation.
-
avalon.pipeline.
loaders_from_representation
(loaders, representation)¶ Return all compatible loaders for a representation.
-
avalon.pipeline.
on
(event, callback)¶ Call callback on event
Register callback to be run when event occurs.
Example
>>> def on_init(): ... print("Init happened") ... >>> on("init", on_init) >>> del on_init
Parameters: - event (str) – Name of event
- callback (callable) – Any callable
-
avalon.pipeline.
plugin_from_module
(superclass, module)¶ Return plug-ins from module
Parameters: - superclass (superclass) – Superclass of subclasses to look for
- module (types.ModuleType) – Imported module from which to parse valid Avalon plug-ins.
Returns: List of plug-ins, or empty list if none is found.
-
avalon.pipeline.
publish
()¶ Shorthand to publish from within host
-
avalon.pipeline.
register_config
(config)¶ Register a new config for the current process
Parameters: config (ModuleType) – A module implementing the Config API.
-
avalon.pipeline.
register_host
(host)¶ Register a new host for the current process
Parameters: host (ModuleType) – A module implementing the Host API interface. See the Host API documentation for details on what is required, or browse the source code.
-
avalon.pipeline.
register_plugin
(superclass, obj)¶ Register an individual obj of type superclass
Parameters: - superclass (type) – Superclass of plug-in
- obj (object) – Subclass of superclass
-
avalon.pipeline.
register_plugin_path
(superclass, path)¶ Register a directory of one or more plug-ins
Parameters: - superclass (type) – Superclass of plug-ins to look for during discovery
- path (str) – Absolute path to directory in which to discover plug-ins
-
avalon.pipeline.
register_root
(path)¶ Register currently active root
-
avalon.pipeline.
registered_config
()¶ Return currently registered config
-
avalon.pipeline.
registered_host
()¶ Return currently registered host
-
avalon.pipeline.
registered_plugin_paths
()¶ Return all currently registered plug-in paths
-
avalon.pipeline.
registered_root
()¶ Return currently registered root
-
avalon.pipeline.
remove
(container)¶ Remove a container
-
avalon.pipeline.
switch
(container, representation)¶ Switch a container to representation
Parameters: - container (dict) – container information
- representation (dict) – representation data from document
Returns: function call
-
avalon.pipeline.
uninstall
()¶ Undo all of what install() did
-
avalon.pipeline.
update
(container, version=-1)¶ Update a container
-
avalon.pipeline.
update_current_task
(task=None, asset=None, app=None)¶ Update active Session to a new task work area.
This updates the live Session to a different asset, task or app.
Parameters: - task (str) – The task to set.
- asset (str) – The asset to set.
- app (str) – The app to set.
Returns: The changed key, values in the current Session.
Return type: dict
Schema¶
Wrapper around jsonschema
Schemas are implicitly loaded from the /schema directory of this project.
-
avalon.schema.
_cache
¶ Cache of previously loaded schemas
- Resources:
- http://json-schema.org/ http://json-schema.org/latest/json-schema-core.html http://spacetelescope.github.io/understanding-json-schema/index.html
-
avalon.schema.
validate
(data, schema=None)¶ Validate data with schema
Parameters: - data (dict) – JSON-compatible data
- schema (str) – DEPRECATED Name of schema. Now included in the data.
Raises: ValidationError on invalid schema –
Inventory¶
Utilities for interacting with database through the Inventory API
Until assets are created entirely in the database, this script provides a bridge between the file-based project inventory and configuration.
- Usage:
$ # Create new project: $ python -m avalon.inventory –init $ python -m avalon.inventory –save
$ # Manage existing project $ python -m avalon.inventory –load $ # Update the .inventory.toml or .config.toml $ python -m avalon.inventory –save
-
avalon.inventory.
init
(name)¶ Initialise a new project called name
Upon creating a new project, call init to establish an example inventory and configuration for your project.
Parameters: name (str) – Name of new project
-
avalon.inventory.
save
(name, config, inventory)¶ Write config and inventory to database as name
Given a configuration and inventory, this function writes the changes to the current database.
Parameters: - name (str) – Project name
- config (dict) – Current configuration
- inventory (dict) – Current inventory
-
avalon.inventory.
load
(name)¶ Read project called name from database
Parameters: name (str) – Project name
I/O¶
Wrapper around interactions with the database
-
class
avalon.io.
ObjectId
(oid=None)¶ A MongoDB ObjectId.
-
binary
¶ 12-byte binary representation of this ObjectId.
-
classmethod
from_datetime
(generation_time)¶ Create a dummy ObjectId instance with a specific generation time.
This method is useful for doing range queries on a field containing
ObjectId
instances.Warning
It is not safe to insert a document containing an ObjectId generated using this method. This method deliberately eliminates the uniqueness guarantee that ObjectIds generally provide. ObjectIds generated with this method should be used exclusively in queries.
generation_time will be converted to UTC. Naive datetime instances will be treated as though they already contain UTC.
An example using this helper to get documents where
"_id"
was generated before January 1, 2010 would be:>>> gen_time = datetime.datetime(2010, 1, 1) >>> dummy_id = ObjectId.from_datetime(gen_time) >>> result = collection.find({"_id": {"$lt": dummy_id}})
Parameters: - generation_time:
datetime
to be used as the generation time for the resulting ObjectId.
- generation_time:
-
generation_time
¶ A
datetime.datetime
instance representing the time of generation for thisObjectId
.The
datetime.datetime
is timezone aware, and represents the generation time in UTC. It is precise to the second.
-
classmethod
is_valid
(oid)¶ Checks if a oid string is valid or not.
Parameters: - oid: the object id to validate
New in version 2.3.
-
-
exception
avalon.io.
InvalidId
¶ Raised when trying to create an ObjectId from invalid data.
-
avalon.io.
install
()¶ Establish a persistent connection to the database
-
avalon.io.
uninstall
()¶ Close any connection to the database
-
avalon.io.
projects
(*args, **kwargs)¶ List available projects
Returns: list of project documents
-
avalon.io.
locate
(path)¶ Traverse a hierarchy from top-to-bottom
Example
representation = locate([“hulk”, “Bruce”, “modelDefault”, 1, “ma”])
Returns: representation (ObjectId)
-
avalon.io.
insert_one
(*args, **kwargs)¶
-
avalon.io.
find
(*args, **kwargs)¶
-
avalon.io.
find_one
(*args, **kwargs)¶
-
avalon.io.
save
(*args, **kwargs)¶ Deprecated, please use replace_one
-
avalon.io.
replace_one
(*args, **kwargs)¶
-
avalon.io.
update_many
(*args, **kwargs)¶
-
avalon.io.
distinct
(*args, **kwargs)¶
-
avalon.io.
drop
(*args, **kwargs)¶
-
avalon.io.
delete_many
(*args, **kwargs)¶
-
avalon.io.
parenthood
(document)¶
Lib¶
Helper functions
-
avalon.lib.
time
()¶ Return file-system safe string of current date and time
-
avalon.lib.
log
(cls)¶ Decorator for attaching a logger to the class cls
Loggers inherit the syntax {module}.{submodule}
- Example
>>> @log ... class MyClass(object): ... pass >>> >>> myclass = MyClass() >>> myclass.log.info('Hello World')